如何使用 Langchain 访问 Yahoo Finance 新闻 API
想要创建由GPT-4o、Claude 3.5 Sonnet、Llama 3.1 405B、Google Gemini Pro等驱动的自动化人工智能代理吗?
接下来,您一定不能错过Anakin AI!使用无代码工作流程,为您定制的智能AI自动化您的工作流程!
立即在这里查看👇👇
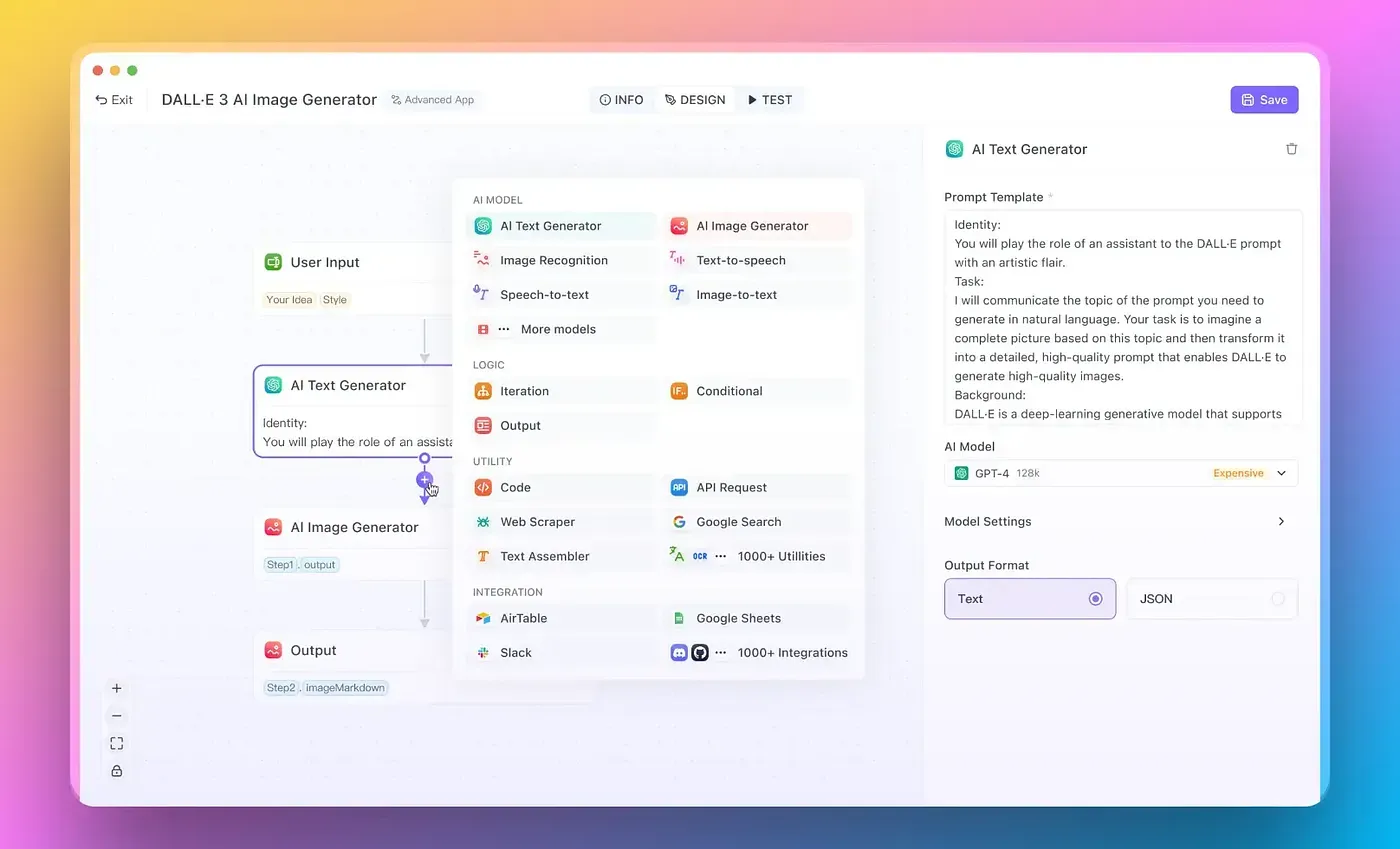
如何使用LangChain访问Yahoo财经新闻API:一个全面指南
了解雅虎财经新闻API:如何使用LangChain访问雅虎财经新闻API
雅虎财经新闻API提供了许多与股票,金融,经济事件和其他相关主题有关的新闻文章和更新。要有效地使用LangChain来处理此API,您必须首先了解其能力和限制。该API允许开发人员根据关键字,时间戳和其他标准获取特定新闻文章,使其成为实时监控财经新闻的强大工具。
要通过LangChain访问Yahoo Finance News API,请确保您拥有一个活跃的Yahoo开发者账户和必要的凭证,通常包括API密钥。这将是在进行API请求时进行身份验证所必需的。
步骤1:获取您的API密钥
- 注册一个Yahoo开发者账号。
- 导航到Yahoo开发人员门户,并创建一个新的应用程序。
- 一旦您的应用程序创建成功,请在应用程序设置中找到您的API密钥。
现在您已经获得了API密钥,是时候设置LangChain以访问Yahoo Finance News API了。
设置开发环境:如何使用LangChain访问Yahoo Finance News API
使用LangChain访问Yahoo Finance新闻API,您需要设置Python开发环境。按照以下步骤进行安装:
步骤2:设置Python环境
- 从官方网站安装Python。
- 创建一个新的虚拟环境:
- python -m venv langchain-env
- 激活虚拟环境:
- 对于Windows:
- langchain-env\Scripts\activate 语言链环境\脚本\激活
- 对于 macOS/Linux:
- 源码 langchain-env/bin/activate
- 安装所需的库:
- pip 安装 langchain 请求
有了环境设置,您可以开始编写代码来访问Yahoo Finance新闻API。
使用LangChain编写代码访问雅虎财经新闻API:如何使用LangChain访问雅虎财经新闻API
下一步是编写代码,利用LangChain向Yahoo Finance News API 发送请求。以下是如何实现这一点的详细示例。
第三步:访问API的代码示例
import os
import requests
from langchain.llms import OpenAI
from langchain.chains import LLMChain
# Set your Yahoo Finance API key
API_KEY = 'YOUR_YAHOO_FINANCE_API_KEY'
BASE_URL = 'https://finance.api.yahoo.com/v1/news'
def fetch_yahoo_finance_news(query, count=10):
url = f"{BASE_URL}?query={query}&count={count}"
headers = {'Authorization': f'Bearer {API_KEY}'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json()
else:
raise Exception(f"Error fetching data: {response.status_code} {response.text}")
def main():
query = input("Enter the stock symbol or topic for news: ")
news_data = fetch_yahoo_finance_news(query)
for article in news_data['articles']:
print(f"Title: {article['title']}")
print(f"Link: {article['link']}\n")
if __name__ == "__main__":
main()
在这段代码中:
- 我们定义一个函数fetch_yahoo_finance_news来处理API请求。
- 主要功能是接受用户输入的股票代码或主题,并打印新闻文章的标题和链接。
处理和分析新闻数据:如何使用LangChain访问Yahoo Finance新闻API
一旦您成功从雅虎财经新闻API中获取新闻数据,了解如何处理和分析这些数据就变得至关重要。LangChain可以进一步整合到您的分析过程中。
第四步:使用LangChain分析新闻文章 您可以利用LangChain的功能从检索到的文章中生成摘要或提取情感。以下是如何总结文章的示例:
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.prompts import ChatPromptTemplate
def summarize_articles(articles):
text_splitter = RecursiveCharacterTextSplitter()
for article in articles['articles']:
title = article['title']
content = article['content']
# Split and summarize the content
text_chunks = text_splitter.split_text(content)
prompt_template = ChatPromptTemplate.from_template(
"Please summarize the following article:\n{content}"
)
for chunk in text_chunks:
summary_prompt = prompt_template.format(content=chunk)
response = llm.generate_response(summary_prompt)
print(f"Summary for '{title}': {response}\n")
if __name__ == "__main__":
query = input("Enter the stock symbol or topic for news: ")
news_data = fetch_yahoo_finance_news(query)
summarize_articles(news_data)
在这个片段中:
- 我们利用LangChain的RecursiveCharacterTextSplitter将文章内容拆分成可管理的文本片段,以便进行摘要。
- ChatPromptTemplate用于向您的语言模型发送结构化请求以进行摘要。
错误处理和日志记录:如何使用LangChain访问Yahoo Finance新闻API
在与API一起工作时,实现错误处理和日志记录是至关重要的,以捕获在请求过程中出现的任何问题。
步骤5:实现错误处理 修改您的fetch_yahoo_finance_news函数以包含错误记录:
import logging
# Set up logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def fetch_yahoo_finance_news(query, count=10):
url = f"{BASE_URL}?query={query}&count={count}"
headers = {'Authorization': f'Bearer {API_KEY}'}
try:
response = requests.get(url, headers=headers)
if response.status_code == 200:
logging.info(f"Successfully fetched news for query: {query}")
return response.json()
else:
logging.error(f"Error fetching data: {response.status_code} {response.text}")
raise Exception(f"Error fetching data: {response.status_code} {response.text}")
except Exception as e:
logging.exception(f"An exception occurred: {str(e)}")
if __name__ == "__main__":
query = input("Enter the stock symbol or topic for news: ")
news_data = fetch_yahoo_finance_news(query)
# Proceed with further processing...
这个经过调整的fetch_yahoo_finance_news版本包括对成功请求和失败的日志信息记录,让您可以随着时间监视系统的行为。
实时更新和自动化:如何通过LangChain访问Yahoo Finance新闻API
为了使您的应用程序保持动态,考虑自动化定期获取和处理新闻数据的过程。这对于交易机器人和金融分析平台尤为重要。
第六步:自动化新闻获取流程 您可以使用类似schedule或APScheduler的库来设置定时任务。以下是一个使用schedule的示例:
import schedule
import time
def job():
query = "AAPL" # Example stock symbol for Apple Inc.
logging.info(f"Fetching news for: {query}")
news_data = fetch_yahoo_finance_news(query)
summarize_articles(news_data)
# Schedule job to run every hour
schedule.every().hour.do(job)
while True:
schedule.run_pending()
time.sleep(1)
通过运行这个计划任务,应用程序将每小时自动获取和处理指定查询的新闻头条,保持您的见解新鲜和及时。
构建用户界面:如何通过LangChain访问Yahoo财经新闻API
虽然命令行应用程序很有用,但用户友好的界面显著提高了可用性。利用诸如Flask或Streamlit之类的工具,您可以创建一个简单的网络应用程序以访问雅虎财经新闻。
这是一个基本的Flask应用程序大纲:
第7步:使用Flask创建一个简单的Web界面
from flask import Flask, render_template, request
import fetch_module
app = Flask(__name__)
@app.route('/', methods=['GET', 'POST'])
def index():
news = []
if request.method == 'POST':
query = request.form['query']
news = fetch_module.fetch_yahoo_finance_news(query)
return render_template('index.html', news=news)
if __name__ == "__main__":
app.run(debug=True)
相应的HTML文件(index.html)可以包括一个简单的表单来输入查询,并显示获取的新闻文章。
这样一来,您的用户可以轻松获取金融新闻和见解,而无需深入研究命令行的复杂性。
通过遵循这些步骤和示例,以及如何使用LangChain访问雅虎财经新闻API,您可以创建一个强大的应用程序,有效地收集和处理与您特定需求相关的财经新闻数据。
想要创建由GPT-4o、克劳德3.5 Sonnet、Llama 3.1 405B、Google Geimini Pro等技术驱动的自动化人工智能代理吗?
接下来,您绝对不能错过Anakin AI!您可以通过无代码工作流自动化您的工作流程,获得定制的代理人人工智能!
现在在这里检查一下👇👇
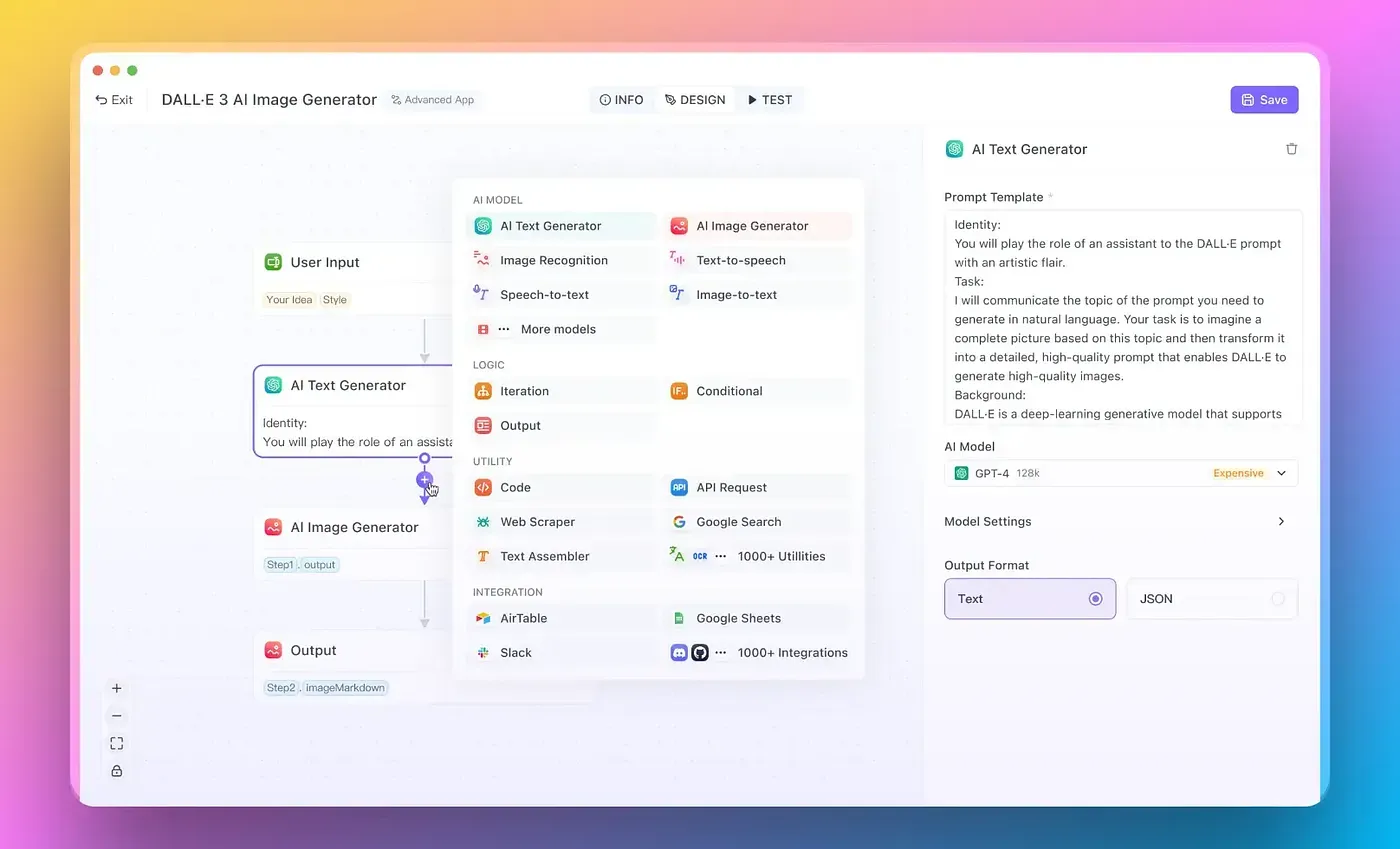