代理商和工具—ReAct 聊天
代理人和工具 - ReAct Chat 是指在会话式人工智能中使用的框架,代理人(通常由语言模型驱动)通过回应用户的查询并利用外部工具来提供更准确和相关的答案。 “ReAct”(Reason and Act)方法不仅允许代理人基于其内部知识生成响应,还可以积极与工具交互,如搜索引擎、API或数据库,以获得实时信息、处理数据或执行特定任务。
在这个框架中,代理被设计为“推理”用户查询,然后通过调用适当的工具来增强响应进行“行动”。对话是有结构的,代理在交互中保持上下文,常常使用记忆机制。这种方法特别适用于复杂情况,其中固定的响应是不够的,代理需要动态访问外部资源来满足用户的请求。
让我们通过一个实际例子来看看这个问题:
- 加载环境变量:
from dotenv import load_dotenv
load_dotenv()
这段代码从.env文件加载环境变量,其中可能包含敏感信息,如API密钥或配置设置。load_dotenv()使这些变量在脚本中可用。
2. 导入所需的库:
from langchain import hub
from langchain.agents import AgentExecutor, create_structured_chat_agent
from langchain.memory import ConversationBufferMemory
from langchain_core.messages import AIMessage, HumanMessage, SystemMessage
from langchain_core.tools import Tool
from langchain_openai import ChatOpenAI
这些导入从langchain库中引入了各种组件,如工具,内存,代理和消息,以及将用于创建会话代理的ChatOpenAI模型。
3. 定义工具:
# Define Tools
def get_current_time(*args, **kwargs):
"""Returns the current time in H:MM AM/PM format."""
import datetime
now = datetime.datetime.now()
return now.strftime("%I:%M %p")
def search_wikipedia(query):
"""Searches Wikipedia and returns the summary of the first result."""
from wikipedia import summary
try:
# Limit to two sentences for brevity
return summary(query, sentences=2)
except:
return "I couldn't find any information on that."
两个功能被定义为代理可以使用的工具:
- 获取当前时间:返回当前时间。
- 搜索维基百科(查询):为给定的查询搜索维基百科,并返回第一个结果的摘要。
4. 为代理定义工具:
# Define the tools that the agent can use
tools = [
Tool(
name="Time",
func=get_current_time,
description="Useful for when you need to know the current time.",
),
Tool(
name="Wikipedia",
func=search_wikipedia,
description="Useful for when you need to know information about a topic.",
),
]
定义的工具被整理成一个列表,其中列出了每个工具的名称,功能和描述。这些工具将在对话过程中供代理使用。
5. 加载 JSON 聊天提示:
prompt = hub.pull("hwchase17/structured-chat-agent")
这一行从模型中心加载了一个结构化的聊天提示,该模型中心定义了与代理进行交互的格式和结构。
6. 初始化语言模型:
# Initialize a ChatOpenAI model
llm = ChatOpenAI(model="gpt-4o-mini")
使用gpt-4o-mini初始化ChatOpenAI模型,该模型将作为在聊天中生成回复的语言模型。
7. 创建对话记忆:
# Create a structured Chat Agent with Conversation Buffer Memory
# ConversationBufferMemory stores the conversation history, allowing the agent to maintain context across interactions
memory = ConversationBufferMemory(
memory_key="chat_history", return_messages=True)
创建一个ConversationBufferMemory对象来存储和管理对话历史,允许代理在多个交互中保持上下文。
8. 创建聊天代理:
# create_structured_chat_agent initializes a chat agent designed to interact using a structured prompt and tools
# It combines the language model (llm), tools, and prompt to create an interactive agent
agent = create_structured_chat_agent(llm=llm, tools=tools, prompt=prompt)
通过结合语言模型(LLM)、定义的工具和结构化聊天提示,初始化一个结构化的聊天代理。这个代理将根据给定的提示和可用工具来处理交互。
9. 创建代理执行器:
# AgentExecutor is responsible for managing the interaction between the user input, the agent, and the tools
# It also handles memory to ensure context is maintained throughout the conversation
agent_executor = AgentExecutor.from_agent_and_tools(
agent=agent,
tools=tools,
verbose=True,
memory=memory, # Use the conversation memory to maintain context
handle_parsing_errors=True, # Handle any parsing errors gracefully
)
AgentExecutor 管理用户、代理人和工具之间的交互。 它通过记忆确保对话上下文得以保持,并处理可能发生的任何解析错误。
10. 添加初始系统消息:
# Initial system message to set the context for the chat
# SystemMessage is used to define a message from the system to the agent, setting initial instructions or context
initial_message = "You are an AI assistant that can provide helpful answers using available tools.\nIf you are unable to answer, you can use the following tools: Time and Wikipedia."
memory.chat_memory.add_message(SystemMessage(content=initial_message))
向会话记忆中添加初始系统消息,为代理设置上下文和指导,告知其角色和可使用的工具。
11. 运行聊天循环:
# Chat Loop to interact with the user
while True:
user_input = input("User: ")
if user_input.lower() == "exit":
break
# Add the user's message to the conversation memory
memory.chat_memory.add_message(HumanMessage(content=user_input))
# Invoke the agent with the user input and the current chat history
response = agent_executor.invoke({"input": user_input})
print("Bot:", response["output"])
# Add the agent's response to the conversation memory
memory.chat_memory.add_message(AIMessage(content=response["output"]))
实现一个循环,用户可以持续与代理互动。用户的输入被添加到对话记忆中,代理的响应被生成并打印。对话会持续直到用户输入“exit”。对话历史保持以为未来互动提供上下文。
这段代码设置了一个会话式AI助手,它可以使用当前时间和维基百科作为工具来回答用户的查询,跨多个交互保持上下文。让我们看看输出是什么样子。
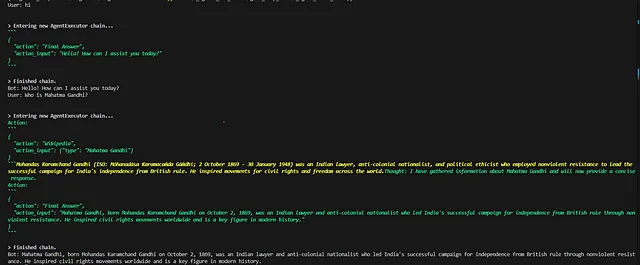
参考资料:
- 保持HTML结构,将以下英文文本翻译成简体中文:https://python.langchain.com/v0.1/docs/modules/agents/
2. https://brandonhancock.io/langchain-master-class 2. https://brandonhancock.io/langchain-master-class
3. https://python.langchain.com/v0.1/docs/modules/tools/
4. https://smith.langchain.com/hub/hwchase17/structured-chat-agent?organizationId=6e7cb68e-d5eb-56c1-8a8a-5a32467e2996 4. https://smith.langchain.com/hub/hwchase17/structured-chat-agent?organizationId=6e7cb68e-d5eb-56c1-8a8a-5a32467e2996