To translate "OpenAI API Integration to React Native App" into simplified Chinese while keeping the HTML structure, you would write: ```html
OpenAI API集成到React Native应用
``` In this HTML snippet: - `` and `
` are used to denote a paragraph in HTML. - "OpenAI API集成到React Native应用" is the translated text in simplified Chinese.在当今的科技领域中,人工智能(AI)和机器学习正在改变我们与技术互动的方式。OpenAI 强大的 API,如 GPT-4,为开发人员提供了构建智能应用程序的令人兴奋的可能性。如果您希望将 OpenAI 的 API 集成到您的 React Native 应用中,本指南将逐步为您介绍整个过程。
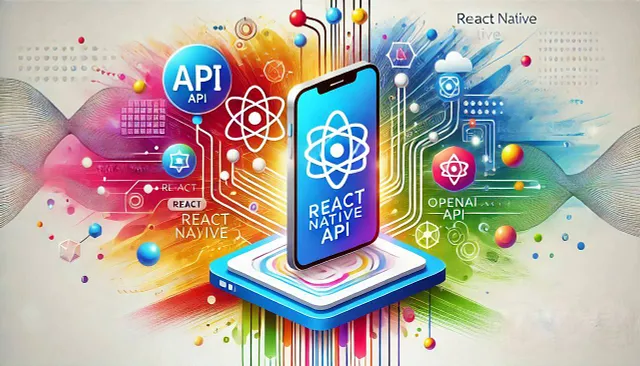
To translate "Prerequisites" to simplified Chinese while keeping HTML structure, you can use the following: ```html
先决条件
``` This HTML snippet maintains the structure while displaying "Prerequisites" in simplified Chinese.在深入整合之前,请确保您具备以下内容:
To translate "Node.js and npm installed: Make sure you have Node.js and npm installed on your machine." into simplified Chinese while keeping HTML structure, you can use the following: ```html Node.js 和 npm 已安装:确保您的计算机上已安装 Node.js 和 npm。 ``` In this translation: - `` tags are used to wrap different parts of the sentence for styling or formatting purposes. - The text inside each `` corresponds to the translated phrases: - "Node.js 和 npm 已安装:" means "Node.js and npm installed:" - "确保您的计算机上已安装 Node.js 和 npm。" means "Make sure you have Node.js and npm installed on your machine." Ensure your HTML document includes appropriate charset meta tag to support Chinese characters, such as ``.
Certainly! Here's the translated text in simplified Chinese: **2. React Native 环境设置:设置您的 React Native 环境。您可以按照官方的 React Native 文档进行操作。**
Sure, here's the text translated into simplified Chinese while maintaining HTML structure: ```html 3. OpenAI API key: 在OpenAI平台上注册并获取您的API密钥。 ``` This HTML snippet preserves the original structure and incorporates the translated text for "Sign up on the OpenAI platform and obtain your API key" in simplified Chinese.
Certainly! Here's the translation of "Step 1: Create a New React Native Project" into simplified Chinese, while keeping the HTML structure: ```html
步骤 1:创建一个新的React Native项目
```Sure, here's the translation in simplified Chinese while keeping the HTML structure: ```html
首先,创建一个新的React Native项目。打开你的终端并运行:
``` This HTML snippet preserves the original English text while providing the translated text in simplified Chinese.npx react-native init OpenAIIntegration
在保持HTML结构的情况下,将以下英文文本翻译为简体中文: 导航至您的项目目录:
cd OpenAIIntegration
Step 2: 安装 Axios
Sure, here's the simplified Chinese translation of the text while maintaining HTML structure: ```html Axios 是一个基于 Promise 的 HTTP 客户端,用于发送请求。使用 npm 安装它: ``` This text translates directly while preserving the HTML structure.
npm install axios
Sure, here's how you can write "Step 3: Create an API Service" in simplified Chinese while keeping the HTML structure: ```html Step 3: 创建 API 服务 ``` In this HTML snippet: - `` specifies that the text inside it is in simplified Chinese. - "Step 3: " remains in English to maintain clarity and consistency with the original structure. - "创建 API 服务" translates to "Create an API Service" in simplified Chinese.
在您的项目目录中创建一个名为 OpenAIService.js 的新文件。此文件将处理与 OpenAI 的 API 请求。
// OpenAIService.js
import axios from 'axios';
const API_KEY = 'your_openai_api_key';
const openAI = axios.create({
baseURL: 'https://api.openai.com/v1',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`,
},
});
export const generateText = async (prompt) => {
try {
const response = await openAI.post('/completions', {
model: 'text-davinci-004',
prompt: prompt,
max_tokens: 150,
});
return response.data.choices[0].text;
} catch (error) {
console.error('Error generating text:', error);
throw error;
}
};
Sure, the translation of "Step 4: Create a User Interface" into simplified Chinese while keeping HTML structure would be: ```html 步骤 4:创建用户界面 ```
在打开 App.js 文件并修改它以创建与 OpenAI API 交互的基本用户界面。
// App.js
import React, { useState } from 'react';
import { SafeAreaView, StyleSheet, TextInput, Button, Text, View } from 'react-native';
import { generateText } from './OpenAIService';
const App = () => {
const [prompt, setPrompt] = useState('');
const [result, setResult] = useState('');
const handleGenerateText = async () => {
try {
const text = await generateText(prompt);
setResult(text);
} catch (error) {
console.error('Error:', error);
}
};
return (
<SafeAreaView style={styles.container}>
<View style={styles.inputContainer}>
<TextInput
style={styles.input}
placeholder="Enter your prompt"
value={prompt}
onChangeText={setPrompt}
/>
<Button title="Generate Text" onPress={handleGenerateText} />
</View>
{result && <Text style={styles.result}>{result}</Text>}
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
padding: 16,
},
inputContainer: {
marginBottom: 24,
},
input: {
borderWidth: 1,
borderColor: '#ccc',
padding: 8,
marginBottom: 8,
},
result: {
marginTop: 24,
fontSize: 16,
},
});
export default App;
Sure, here is the translation of "Step 5: Test Your Application" in simplified Chinese, keeping the HTML structure: ```html Step 5: 测试您的应用程序 ``` This HTML code ensures the text "测试您的应用程序" (which means "Test Your Application" in simplified Chinese) is displayed correctly while indicating the language for proper rendering and accessibility.
Here's the translation while keeping the HTML structure: ```html 运行您的 React Native 应用程序以确保一切正常工作: ```
npx react-native run-android
# or
npx react-native run-ios
Sure, here's the HTML structure with the text translated into simplified Chinese: ```html
在文本输入框中输入提示,然后点击“生成文本”按钮。您应该能够在屏幕上看到来自OpenAI API的响应。
``` This HTML snippet includes the translated text: - "Enter a prompt in the text input field and press the “Generate Text” button." translates to "在文本输入框中输入提示,然后点击“生成文本”按钮。" - "You should see the response from the OpenAI API displayed on the screen." translates to "您应该能够在屏幕上看到来自OpenAI API的响应。"Here is the translation with HTML structure intact: ```html
结论
``````html
将 OpenAI 的 API 集成到 React Native 应用中可以显著提升用户体验,通过提供智能的响应和互动。本文指南涵盖了设置和集成 OpenAI API 的基本步骤。基于这些基础,您可以探索更多高级功能,创建更强大的应用程序。
```Sure, here's the translated text in simplified Chinese: 关于OpenAI API的更多信息,请参考官方文档。祝编码愉快!