To keep the HTML structure intact while translating "Effectively Analyze PDFs with GPT-4o API" into simplified Chinese, you can use the following code snippet: ```html 有效地使用GPT-4o API分析PDF文件 ``` This HTML snippet ensures that the translated Chinese text ("有效地使用GPT-4o API分析PDF文件") is correctly marked as simplified Chinese (`zh-CN`) within the HTML document.
在使用GPT-4o API分析PDF文件时,用户经常会面临几个问题和挑战。
Sure, here is the translation of the text into simplified Chinese while keeping the HTML structure: ```html
在Web GUI(chatgpt.com)中,用户只需拖放PDF并在提示中提出问题,但是使用API则需要您多做一些额外步骤:
```- Sure, here's the translation of the provided text into simplified Chinese while keeping the HTML structure: ```html 创建(或获取)Assistant对象。Assistant对象必须启用工具下的文件搜索功能。请注意,您无法启用JSON模式,因为它对于“file_search”不可用(JSON模式仅适用于“function”工具)。 ```
- Sure, here's the translation in simplified Chinese within an HTML structure: ```html 上传文件 — 一个PDF文件,或其他支持的格式。您需要保存文件的ID,以便稍后创建线程时传递它。请注意,.pdf将被处理为文本文档,而不是图像(因此成本较低)。 ```
- Sure, here's the HTML structure with the translated text in simplified Chinese:
```html
Translate Text to Chinese 创建一个主题,向其添加一个包含文件附件和提示数据的“用户”消息,并将助理分配给它。
``` In simplified Chinese, the translated text is: "创建一个主题,向其添加一个包含文件附件和提示数据的“用户”消息,并将助理分配给它。" This HTML structure ensures the text is displayed correctly and maintains proper encoding for Chinese characters. - Sure, here's the translation in simplified Chinese while keeping the HTML structure: ```html 运行线程。我们将以轮询模式(而非流模式)运行它,因此该函数仅在运行生命周期结束后才会返回(无论是完成、失败、被取消或过期)。 ``` This HTML structure preserves the original formatting and text content while presenting the translation in simplified Chinese.
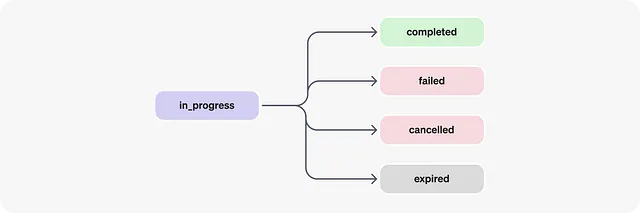
Certainly! Here's the translated text in simplified Chinese, keeping the HTML structure: ```html 5. 如果您想获得 JSON 输出,您需要手动解析 GPT-4o 的响应。模型会返回类似以下文本的内容,因此您需要使用正则表达式(或类似方法)提取有效的 JSON 输出。 ``` This HTML code maintains the original structure while presenting the translated text in simplified Chinese.
Of course, here's the total purchase order amount:
```json
{
"price": 1234.56
}
```
To translate the English text to simplified Chinese while keeping the HTML structure intact, you can use the following: ```html 6. (可选)删除您上传的文件。OpenAI API文档提到每个文件的大小可以高达512MB,并且所有文件(在一个组织中)的总大小必须低于100GB。 ``` In this translation: - **(可选)** means "(optional)". - **删除您上传的文件** means "delete the file(s) you have uploaded". - **OpenAI API文档** translates to "OpenAI API documentation". - **每个文件的大小可以高达512MB** translates to "each file can be up to 512MB in size". - **所有文件(在一个组织中)的总大小必须低于100GB** means "all files together (in an organization) have to be below 100GB".
Sure, here is the translation of "The code" into simplified Chinese while keeping the HTML structure intact: ```html 代码 ``` In this example: - `` is used to mark up the text as Chinese. - `lang="zh-CN"` attribute specifies the language as simplified Chinese. - `代码` is the translated text for "The code".
To translate the English text to simplified Chinese while keeping the HTML structure intact, you can use the following: ```html
这是实现此功能的Python代码,使用版本1.33.0的openai库(助手仍处于Beta版):
``` This HTML snippet will display the translated Chinese text in a paragraph (``) element. Just replace the English text with the translated Chinese text within the `
` tags to maintain the HTML structure.
from openai import OpenAI # Version 1.33.0
from openai.types.beta.threads.message_create_params import Attachment, AttachmentToolFileSearch
import json
MY_OPENAI_KEY = 'sk-...' # Add your OpenAI API key
client = OpenAI(api_key=MY_OPENAI_KEY)
# Upload your pdf(s) to the OpenAI API
file = client.files.create(
file=open('my_file.pdf', 'rb'),
purpose='assistants'
)
# Create thread
thread = client.beta.threads.create()
# Create an Assistant (or fetch it if it was already created). It has to have
# "file_search" tool enabled to attach files when prompting it.
def get_assistant():
for assistant in client.beta.assistants.list():
if assistant.name == 'My Assistant Name':
return assistant
# No Assistant found, create a new one
return client.beta.assistants.create(
model='gpt-4o',
description='You are a PDF retrieval assistant.',
instructions="You are a helpful assistant designed to output only JSON. Find information from the text and files provided.",
tools=[{"type": "file_search"}],
# response_format={"type": "json_object"}, # Isn't possible with "file_search"
name='My Assistant Name',
)
# Add your prompt here
prompt = "What's the total price of the purchase order in PDF? Output in JSON format, like:\n{'price': 123.45}"
client.beta.threads.messages.create(
thread_id = thread.id,
role='user',
content=prompt,
attachments=[Attachment(file_id=file.id, tools=[AttachmentToolFileSearch(type='file_search')])]
)
# Run the created thread with the assistant. It will wait until the message is processed.
run = client.beta.threads.runs.create_and_poll(
thread_id=thread.id,
assistant_id=get_assistant().id,
timeout=300, # 5 minutes
# response_format={"type": "json_object"}, # Isn't possible
)
# Eg. issue with openai server
if run.status != "completed":
raise Exception('Run failed:', run.status)
# Fetch outputs of the thread
messages_cursor = client.beta.threads.messages.list(thread_id=thread.id)
messages = [message for message in messages_cursor]
message = messages[0] # This is the output from the Assistant (second message is your message)
assert message.content[0].type == "text"
# Output text of the Assistant
res_txt = message.content[0].text.value
# Because the Assistant can't produce JSON (as we're using "file_search"),
# it will likely output text + some JSON code. We can parse and extract just
# the JSON part, and ignore everything else (eg. gpt4o will start with something
# similar to "Of course, here's the parsed text: {useful_JSON_here}")
if res_txt.startswith('```json'):
res_txt = res_txt[6:]
if res_txt.endswith('```'):
res_txt = res_txt[:-3]
res_txt = res_txt[:res_txt.rfind('}')+1]
res_txt = res_txt[res_txt.find('{'):]
res_txt.strip()
# Parse the JSON output
data = json.loads(res_txt)
print(data)
# Delete the file(s) afterward to preserve space (max 100gb/company)
delete_ok = client.files.delete(file.id)