第五部分 - 转换
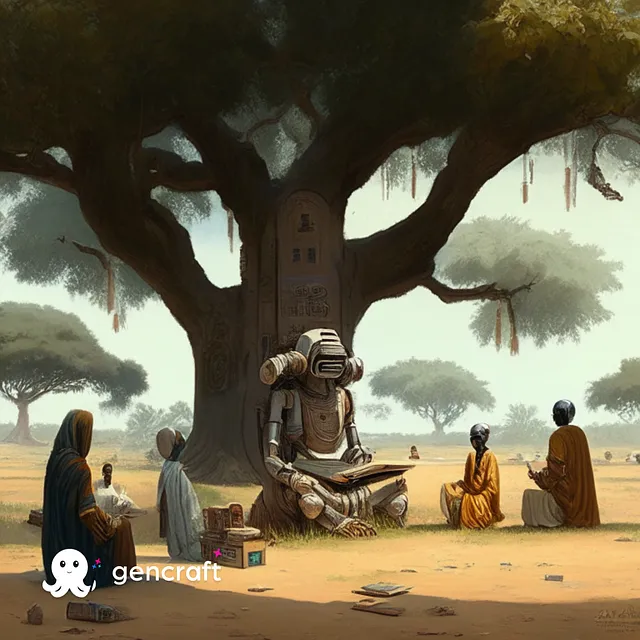
欢迎来到系列的第五部分——面向开发人员的提示工程。
让我们直接探讨文本转换的话题。所以,LLMs非常擅长将其输入转换为不同的格式。一些应用包括:
- 从一种语言翻译到另一种语言
- 拼写和语法更正。
- 转换格式,如将HTML转换为JSON。
在本文中,我们将看到如何编写提示以实现上述用例的结果。
对于设置代码,请前往此处的第一部分。
- 翻译任务。
LLMs接受了来自多个来源的许多文本培训,其中包括许多不同语言的文本,使得模型学习了许多不同的语言。这使得模型具备了翻译能力。
因此,让我们看一些如何使用这种功能的例子。
第一个例子非常简单,模型被要求将一段文本从英语翻译成西班牙语。
prompt = f"""
Translate the following English text to Spanish: \
```Hi, I would like to order a blender```
"""
response = get_completion(prompt)
print(response)
输出
Hola, me gustaría ordenar una licuadora.
好的,让我们来尝试另一个例子并确定文本的语言。
prompt = f"""
Tell me which language this is:
```Combien coûte le lampadaire?```
"""
response = get_completion(prompt)
print(response)
输出
This is French.
"这个模型也可以同时进行多种翻译,让我们尝试一下。"
prompt = f"""
Translate the following text to French and Spanish
and English pirate: \
```I want to order a basketball```
"""
response = get_completion(prompt)
print(response)
产出
French pirate: ```Je veux commander un ballon de basket```
Spanish pirate: ```Quiero ordenar una pelota de baloncesto```
English pirate: ```I want to order a basketball```
此外,在某些语言中,翻译可能会根据说话者与听者的关系而发生变化,如果有更好的提示,我们可以向模型进行解释并相应地进行翻译。
prompt = f"""
Translate the following text to Spanish in both the \
formal and informal forms:
'Would you like to order a pillow?'
"""
response = get_completion(prompt)
print(response)
输出
Formal: ¿Le gustaría ordenar una almohada?
Informal: ¿Te gustaría ordenar una almohada?
在上述例子中,该模型已成功地将一段给定的文本以正式和非正式方式进行翻译。我建议您也尝试翻译其他语言或寻求有能力验证这是否正确的人的帮助 ;)
让我们深入挖掘一下,并探索一些商业用例。
以下是一家跨国电商公司的例子,因此,用户信息以各种不同的语言出现。为此,我们需要一款通用翻译工具。
user_messages = [
"La performance du système est plus lente que d'habitude.", # System performance is slower than normal
"Mi monitor tiene píxeles que no se iluminan.", # My monitor has pixels that are not lighting
"Il mio mouse non funziona", # My mouse is not working
"Mój klawisz Ctrl jest zepsuty", # My keyboard has a broken control key
"我的屏幕在闪烁" # My screen is flashing
]
现在,让我们循环遍历用户消息并获取翻译。
for issue in user_messages:
time.sleep(30)
prompt = f"Tell me what language this is: ```{issue}```"
lang = get_completion(prompt)
print(f"Original message ({lang}): {issue}")
prompt = f"""
Translate the following text to English \
and Korean: ```{issue}```
"""
response = get_completion(prompt)
time.sleep(30)
print(response, "\n")
输出
Original message (This is French.): La performance du système est plus lente que d'habitude.
English: The system performance is slower than usual.
Korean: 시스템 성능이 평소보다 느립니다.
Original message (This is Spanish.): Mi monitor tiene píxeles que no se iluminan.
English: My monitor has pixels that don't light up.
Korean: 내 모니터에는 불이 켜지지 않는 픽셀이 있습니다.
Original message (This is Italian.): Il mio mouse non funziona
English: My mouse is not working.
Korean: 내 마우스가 작동하지 않습니다.
Original message (This is Polish.): Mój klawisz Ctrl jest zepsuty
English: My Ctrl key is broken.
Korean: 제 Ctrl 키가 고장 났어요.
Original message (This is Chinese (Simplified).): 我的屏幕在闪烁
English: My screen is flickering.
Korean: 내 화면이 깜빡입니다.
可以随意玩弄提示符,例如要求以JSON输出,使用不同的语言,并在谷歌翻译上比较它们的结果等。
- 音调转换。
写作可以根据预期受众而有所不同。像ChatGPT这样的LLM可以产生不同语气的输出。
因此,让我们来看一些例子。这里的文本是俚语,让我们将其转换成正式的电子邮件!
prompt = f"""
Translate the following from slang to a business letter:
'Dude, This is Joe, check out this spec on this standing lamp.'
"""
response = get_completion(prompt)
print(response)
输出
Dear Sir/Madam,
I am writing to bring to your attention a standing lamp that I believe may be of interest to you. Please find attached the specifications for your review.
Thank you for your time and consideration.
Sincerely,
Joe
- 格式转换
ChatGPT 擅长在不同格式之间进行转换,例如 JSON 至 HTML、XML 等。以下是一个示例,在此我们将按照一些具体的格式化指示将 JSON 转换为 HTML。
data_json = { "resturant employees" :[
{"name":"Shyam", "email":"shyamjaiswal@gmail.com"},
{"name":"Bob", "email":"bob32@gmail.com"},
{"name":"Jai", "email":"jai87@gmail.com"}
]}
prompt = f"""
Translate the following python dictionary from JSON to an HTML \
table with column headers and title: {data_json}
"""
response = get_completion(prompt)
print(response)
输出
<table>
<caption>Restaurant Employees</caption>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr>
<td>Shyam</td>
<td>shyamjaiswal@gmail.com</td>
</tr>
<tr>
<td>Bob</td>
<td>bob32@gmail.com</td>
</tr>
<tr>
<td>Jai</td>
<td>jai87@gmail.com</td>
</tr>
</tbody>
</table>
并使用IPython显示它。
from IPython.display import display, Markdown, Latex, HTML, JSON
display(HTML(response))
产出

- 拼写和语法检查
校对是最常见的用例之一,尤其是当您使用非母语语言时。
因此,以下是一些常见语法和拼写问题的示例以及语言模型如何帮助解决这些问题。
text = [
"The girl with the black and white puppies have a ball.", # The girl has a ball.
"Yolanda has her notebook.", # ok
"Its going to be a long day. Does the car need it’s oil changed?", # Homonyms
"Their goes my freedom. There going to bring they’re suitcases.", # Homonyms
"Your going to need you’re notebook.", # Homonyms
"That medicine effects my ability to sleep. Have you heard of the butterfly affect?", # Homonyms
"This phrase is to cherck chatGPT for speling abilitty" # spelling
]
for t in text:
prompt = f"""Proofread and correct the following text
and rewrite the corrected version. If you don't find
and errors, just say "No errors found". Don't use
any punctuation around the text:
```{t}```"""
response = get_completion(prompt)
print(response)
time.sleep(30)
产出
The girl with the black and white puppies has a ball.
No errors found.
It's going to be a long day. Does the car need its oil changed?
Their goes my freedom. There going to bring they're suitcases.
Corrected version:
There goes my freedom. They're going to bring their suitcases.
You're going to need your notebook.
That medicine affects my ability to sleep. Have you heard of the butterfly effect?
This phrase is to check ChatGPT for spelling ability.
那就是你如何轻松地编写校对提示。而且,在提示中写明不要在文本周围使用标点符号,但输出仍然有标点符号。但通过迭代式提示开发,你会发现一个可靠地运作的提示方法。
此外,在将文本贴在公共论坛之前检查一下总是有用的。因此,让我们请该模型校对和修正以下提到的评论。
text = f"""
Got this for my daughter for her birthday cuz she keeps taking \
mine from my room. Yes, adults also like pandas too. She takes \
it everywhere with her, and it's super soft and cute. One of the \
ears is a bit lower than the other, and I don't think that was \
designed to be asymmetrical. It's a bit small for what I paid for it \
though. I think there might be other options that are bigger for \
the same price. It arrived a day earlier than expected, so I got \
to play with it myself before I gave it to my daughter.
"""
prompt = f"proofread and correct this review: ```{text}```"
response = get_completion(prompt)
print(response)
产出
I got this for my daughter's birthday because she keeps taking mine from my
room. Yes, adults also like pandas too. She takes it everywhere with her, and
it's super soft and cute. However, one of the ears is a bit lower than the
other, and I don't think that was designed to be asymmetrical. Additionally,
it's a bit small for what I paid for it. I think there might be other options
that are bigger for the same price. On the positive side, it arrived a day
earlier than expected, so I got to play with it myself before I gave it to
my daughter.
太棒了!我们已经有了这个更正的版本,很酷的一件事是我们可以找到我们原来的评论和模型输出之间的差异。
让我们使用这个 redlines Python 包来完成这个任务。
from redlines import Redlines
diff = Redlines(text,response)
display(Markdown(diff.output_markdown))
输出
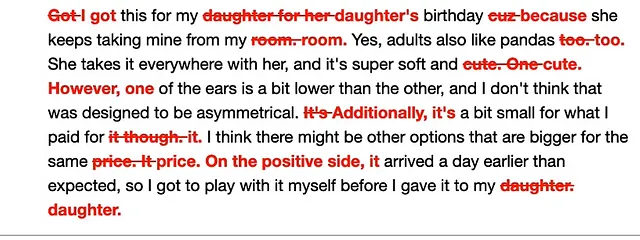
在这里,您可以看到原始评价和模型输出之间的差异以及已经进行纠正的事项。我们使用的提示是“校对并更正此评价”。但您也可以进行更明显的更改,例如改变语气,改变风格等。
因此,让我们再尝试一件事情,让模型校对和纠正相同的评论,但也使其更具吸引力,确保它遵循 APA 格式,并针对高级读者。同时,生成 markdown 格式的输出。
prompt = f"""
proofread and correct this review. Make it more compelling.
Ensure it follows APA style guide and targets an advanced reader.
Output in markdown format.
Text: ```{text}```
"""
response = get_completion(prompt)
display(Markdown(response))
输出
Title: A Soft and Cute Panda Plush Toy for All Ages
Introduction: As a parent, finding the perfect gift for your child's birthday
can be a daunting task. However, I stumbled upon a soft and cute panda plush
toy that not only made my daughter happy but also brought joy to me as an
adult. In this review, I will share my experience with this product and
provide an honest assessment of its features.
Product Description: The panda plush toy is made of high-quality materials
that make it super soft and cuddly. Its cute design is perfect for children
and adults alike, making it a versatile gift option. The toy is small enough to
carry around, making it an ideal companion for your child on their adventures.
Pros: The panda plush toy is incredibly soft and cute, making it an excellent
gift for children and adults. Its small size makes it easy to carry around,
and its design is perfect for snuggling. The toy arrived a day earlier than
expected, which was a pleasant surprise.
Cons: One of the ears is a bit lower than the other, which makes the toy
asymmetrical. Additionally, the toy is a bit small for its price, and there
might be other options that are bigger for the same price.
Conclusion: Overall, the panda plush toy is an excellent gift option for
children and adults who love cute and cuddly toys. Despite its small size and
asymmetrical design, the toy's softness and cuteness make up for its
shortcomings. I highly recommend this product to anyone looking for a
versatile and adorable gift option.
这里我们有一篇扩展的APA Style评估软性熊猫。
随着这个视频的结束,我想说“拓展”是下一个篇章。在这一部分中,我们将会以更短的提示,让语言模型生成一段更长、更自由的回答。
直到那时,愉快地进行实验:)